User manual¶
Installation¶
First, clone the git repository in a directory of your choice using a Command Prompt window:
$ ~\directory-of-my-choice> git clone https://github.com/tum-ens/pyGRETA.git
We recommend using conda and installing the environment from the file ren_ts_new.yml
that you can find in the repository. In the Command Prompt window, type:
$ cd pyGRETA\env\
$ conda env create -f ren_ts_new.yml
Then activate the environment:
$ conda activate ren_ts_new
In the folder code
, you will find multiple files:
File |
Description |
---|---|
config.py |
used for configuration, see below. |
runme.py |
main file, which will be run later using |
lib\initialization.py |
used for initialization. |
lib\input_maps.py |
used to generate input maps for the scope. |
lib\potential.py |
contains functions related to the potential estimation. |
lib\time_series.py |
contains functions related to the generation of time series. |
lib\regression.py |
contains functions related to the regression. |
lib\spatial_functions.py |
contains helping functions related to maps, coordinates and indices. |
lib\physical_models.py |
contains helping functions for the physical/technological modeling. |
lib\correction_functions.py |
contains helping functions for data correction/cleaning. |
lib\util.py |
contains minor helping functions and the necessary python libraries to imported. |
config.py¶
This file contains the user preferences, the links to the input files, and the paths where the outputs should be saved. The paths are initialized in a way that follows a particular folder hierarchy. However, you can change the hierarchy as you wish.
runme.py¶
runme.py
calls the main functions of the code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 | import lib.correction_functions as cf
import lib.spatial_functions as sf
import lib.input_maps as im
import lib.potential as pl
from lib.log import logger
import initialization as ii
import lib.time_series as ts
import lib.regression as rg
import os
import psutil
if __name__ == "__main__":
# logger.setLevel(logging.DEBUG) # Comment out to get more information on the console
if psutil.virtual_memory().available > 50*10**9: # Check if memory size is large enough for multiprocessing
multiprocessing = True
else:
multiprocessing = False
logger.info('Multiprocessing: ' + str(multiprocessing))
configs = sorted(os.listdir('../configs'))
for config in configs: # Iterate over all config files for each country in folder 'configs'
try: # only interrupt current country run in case of failure
logger.info('Started: ' + str(config))
paths, param = ii.initialization(config) # Initialize for each country with the corresponding config defined in folder 'configs'
im.downloadGWA(paths, param) # Download wind speed data from Global Wind Atlas
im.generate_maps_for_scope(paths, param, multiprocessing) # Generate input raster maps
cf.generate_wind_correction(paths, param)
for tech in param["technology"]:
logger.info("Tech: " + tech)
if tech == "Biomass":
im.generate_livestock(paths,param)
pl.generate_biomass_production(paths, param, tech)
pl.report_biomass_potentials(paths, param, tech)
else:
# Generate potential maps and reports
pl.calculate_full_load_hours(paths, param, tech, multiprocessing)
pl.mask_potential_maps(paths, param, tech)
pl.weight_potential_maps(paths, param, tech)
pl.report_potentials(paths, param, tech)
# Generate time series
# ts.find_representative_locations(paths, param, tech)
# ts.generate_time_series_for_representative_locations(paths, param, tech)
# ts.generate_time_series_for_specific_locations(paths, param, tech)
# for tech in param["technology"]:
# logger.info("Tech: " + tech)
# Generate regression coefficients for FLH and TS model matching
# rg.get_regression_coefficients(paths, param, tech)
# Generate times series for combinations of technologies and locations
# ts.generate_time_series_for_regions(paths, param, tech)
except Exception:
logger.info("General exception noted!", exc_info=True)
|
Recommended input sources¶
For a list of GIS data sources, check this wikipedia article.
Weather data from MERRA-2¶
The most important inputs within this model are the weather time series. These are taken from the Modern-Era Retrospective Analysis for Research and Applications, version 2 (MERRA-2), which is the latest atmospheric reanalysis of the modern satellite era produced by NASA’s Global Modeling and Assimilation Office (GMAO) [5]. The parameters taken from MERRA-2 are:
Global Horizontal Irradiance (GHI): Downward shortwave radiation received by a surface horizontal to the ground (SWGDN in MERRA-2 nomenclature).
Top of the Atmosphere Irradiance (TOA): Downward shortwave radiation at the top of the atmosphere (SWTDN in MERRA-2 nomenclature).
Air temperature 2 meters above the ground (T2M).
Northward wind velocity at 50 meters (V50M).
Eastward wind velocity at 50 meters (U50M).
The GHI and TOA data are time-averaged hourly values given in W/m while T2M data are instantaneous values in Kelvin. V50M and U50M are instantaneous hourly values given in m/s.
The spatial arrangement of the data consists of a global horizontal grid structure with a resolution of 576 points in the longitudinal direction and 361 points in the latitudinal direction, resulting in pixels of 5/8° longitude and 1/2° latitude [1].
It is possible to download MERRA-2 dataset for the whole globe or just for a subset of your region of interest. Depending on the MERRA_coverage parameter in config.py, the script can accept both datasets. Note that downloading the coverage for the whole globe is easier but will require a significant amount of space on your drive (coverage of the whole globe requires 13.6 Gb for one year).
In both cases, please follow these instructions to download the MERRA-2 dataset:
In order to download MERRA-2 data using the FTP server, you first need to create an Eathdata account (more on that on their website).
Navigate to the link for the FTP sever here.
In Data Product, choose tavg1_2d_slv_NX and select the Parameters T2M, U50M, V50M to downaload the temperature and the wind speed datasets.
In Spatial Search, enter the coordinates of the bounding box around your region of interest or leave the default values for the whole globe. To avoid problems at the edge of the MERRA-2 cells, use the following set of formulas:
\[\begin{split}\begin{align*} minLat &= \left\lfloor\dfrac{s+0.25}{0.5}\right\rfloor \cdot 0.5 - \epsilon \\ maxLat &= \left\lceil\dfrac{n-0.25}{0.5}\right\rceil \cdot 0.5 + \epsilon \\ minLon &= \left\lfloor\dfrac{w+0.3125}{0.625}\right\rfloor \cdot 0.625 - \epsilon \\ maxLon &= \left\lceil\dfrac{e-0.3125}{0.625}\right\rceil \cdot 0.625 + \epsilon \end{align*}\end{split}\]where [s n w e] are the southern, northern, western, and eastern bounds of the region of interest, which you can read from the shapefile properties in a GIS software, and \(\\epsilon\) a small number.
In Temporal Order Option, choose the year(s) of interest.
Leave the other fields unchanged (no time subsets, no regridding, and NetCDF4 for the output file format).
Repeat the steps 4-6 for the Data Product tavg1_2d_rad_Nx, for which you select the Parameters SWGDN and SWTDN, the surface incoming shortwave flux and the top of the atmosphere incoming shortwave flux.
Follow the instructions in the website to actually download the NetCDF4 files from the urls listed in the text files you obtained.
If you follow these steps to download the data for the year 2015, you will obtain 730 NetCDF files, one for each day of the year and for each data product.
Raster of Mean Wind Speed¶
High resolution (250m) country-wise rasters of mean wind speed from Global wind atlas website will be automatically downloaded by the tool.
Raster of land use¶
Another important input for this model is the land use type. A land use map is useful in the sense that other parameters can be associated with different landuse types, namely:
Urban areas
Ross coefficients
Hellmann coefficients
Albedo
Suitability
Installation cost
etc.
For each land use type, we can assign a value for these parameters which affect
the calculations for solar power and wind speed correction.
The global land use raster for which lib.input_maps.generate_landuse
has been written can be downloaded from the ESA CCI website.
However, this new version requires additional data processing.
The spatial resolution of the land use raster downloaded is 300m, but the resolution used in the model is 250m.
So the landuse raster should be resampled in a GIS software. QGIS can be used easily for doing this.
Shapefile of the region of interest¶
The strength of the tool relies on its versatility, since it can be used for any user-defined regions provided in a shapefile. If you are interested in administrative divisions, you may consider downloading the shapefiles from the website of the Global Administration Divisions (GADM). You can also create your own shapefiles using a GIS software.
Warning
In any case, you need to have at least one attribute called NAME_SHORT containing a string (array of characters) designating each sub-region.
Shapefile of countries¶
A shapefile of all the countries of the world is also needed. It can be downloaded again from GADM. The attribute “GID_0” contains the ISO 3166-1 Alpha-3 codes of the countries, and is currently hard coded in the script.
Warning
If you want to use another source or other code names, you need to edit the name of the attribute “GID_0” and the dictionary dict_countries.csv.
Shapefile of Exclusive Economic Zones (EEZ)¶
A shapefile of the maritime boundaries of all countries is available at the website of the Flanders Marine Institute (VLIZ). It is used to identify offshore areas.
Shapefile of Internal Waters¶
A shapefile of the internal waters boundaries of all countries is available at the website of the Flanders Marine Institute (VLIZ). It is used to identify offshore areas.
Raster of topography / elevation data¶
A high resolution raster (15 arcsec = 1/240° longitude and 1/240° latitude) made of 24 tiles can be downloaded from viewfinder panoramas. Multiple files will be downloaded from this source. They can all be merged and resampled to the resolution of the model (250m) using QGIS, similar to the landuse raster.
Raster of bathymetry¶
A high resolution raster (60 arcsec) of bathymetry can be downloaded from the website of the National Oceanic and Atmospheric Administration (NOAA). The one used in the database is ETOPO1 Ice Surface, cell-registered.
Shapefile of protected areas¶
Any database for protected areas can be used with this tool, in particular the World Database on Protected Areas
published by the International Union for Conservation of Nature (IUCN).
The shapefile has many attributes, but only one is used in the tool: “IUCN_CAT”. If another database is used, an
equivalent attribute with the different categories of the protection has to be used and config.py
has to be updated accordingly.
Airports Coordinates¶
List of airports around the world can be downloaded as a csv file from open data (openflights).
Shapefiles from OSM data¶
Open Street Map data can be downloaded as shapefiles from geofabrik.
The shapefiles for roads, railways and landuse are used in this model.
These shapefiles have many attributes, but only one is used in the tool: “fclass”.
For roads shapefile, the “fclass” types “Motoways, motorways_link, primary, primary-link, secondary, secondary-link, trunk, trunk-link” are filtered prior to using the model.
For railways shapefile, no filtering is necessary.
For landuse shapefile, the “fclass” types “commercial, industrial, quarry, military, park, recreation_ground” are filtered prior to using the model.
If another exclusion criteria is used, config.py
has to be updated accordingly.
Raster of Settlement Footprint¶
A high resolution raster (0.32 arcsec or 10m) of World settlement footprint can be downloaded from open source. The downloaded multiple files need to be merged and resampled to the desired resolution (250m) of the model prior to the run.
Shapefile of HydroLakes¶
Any database for Lakes can be used with this tool, in particular from HydroSheds. No preprocessing is necessary for this dataset.
Shapefile of HydroRivers¶
Any database for Rivers can be used with this tool, in particular from HydroSheds. No preprocessing is necessary for this dataset.
Data of Crop Production¶
Annual crop production data of all countries in the world can be downloaded from the website of Food and Agriculture Organization of United States (FAOSTAT). While downloading, the latest year and “Production Quantity” should be selected as filters.
Data of Forestry Production¶
Annual forestry production data of all countries in the world can be downloaded from the website of Food and Agriculture Organization of United States (FAOSTAT). While downloading, the latest year and “Production Quantity” should be selected as filters.
Shapefile of Livestock density¶
Any dataset for Livestock density can be used with this tool, in particular the rasters created from the data of Food and Agriculture Organization of United States for various animals (FAO GLW3). These files are available at high resolution (5 arc-minutes). The model can read these high resolution rasters and resample them to the resolution of the model.
Recommended workflow¶
The script is designed to be modular and split into four main modules: lib.input_maps
, lib.potential
, lib.time_series
, and lib.regression
.
Warning
The outputs of each module serve as inputs to the following module. Therefore, the user will have to run the script sequentially.
The recommended use cases of each module will be presented in the order in which the user will have to run them.
The use cases associated with each module with examples of their outputs are presented below.
It is recommended to thoroughly read through the configuration file config.py and modify the input paths and computation parameters before starting the runme.py script. Once the configuration file is set, open the runme.py file to define what use case you will be using the script for.
Input raster maps¶
The lib.input_maps
module is used to generate data (mostly raster maps, but also arrays in MAT files) for the spatial scope defined by the user. These data sets include:
Weather data
Land and sea masking
Bathymetry
Topography
Slope
Area
Land use and buffer masking
Protected areas and their buffer masking
Boarder Buffer masking
Airports and buffer masking
Roads Buffer masking
Railway lines Buffer masking
OSM defined areas like mining, military zones Buffer masking
Settlement regions Buffer masking
HydroLakes Buffer masking
HydroRivers Buffer masking
Livestock density
All these maps are needed before the potential or time series modules can be used for a specific spatial scope.
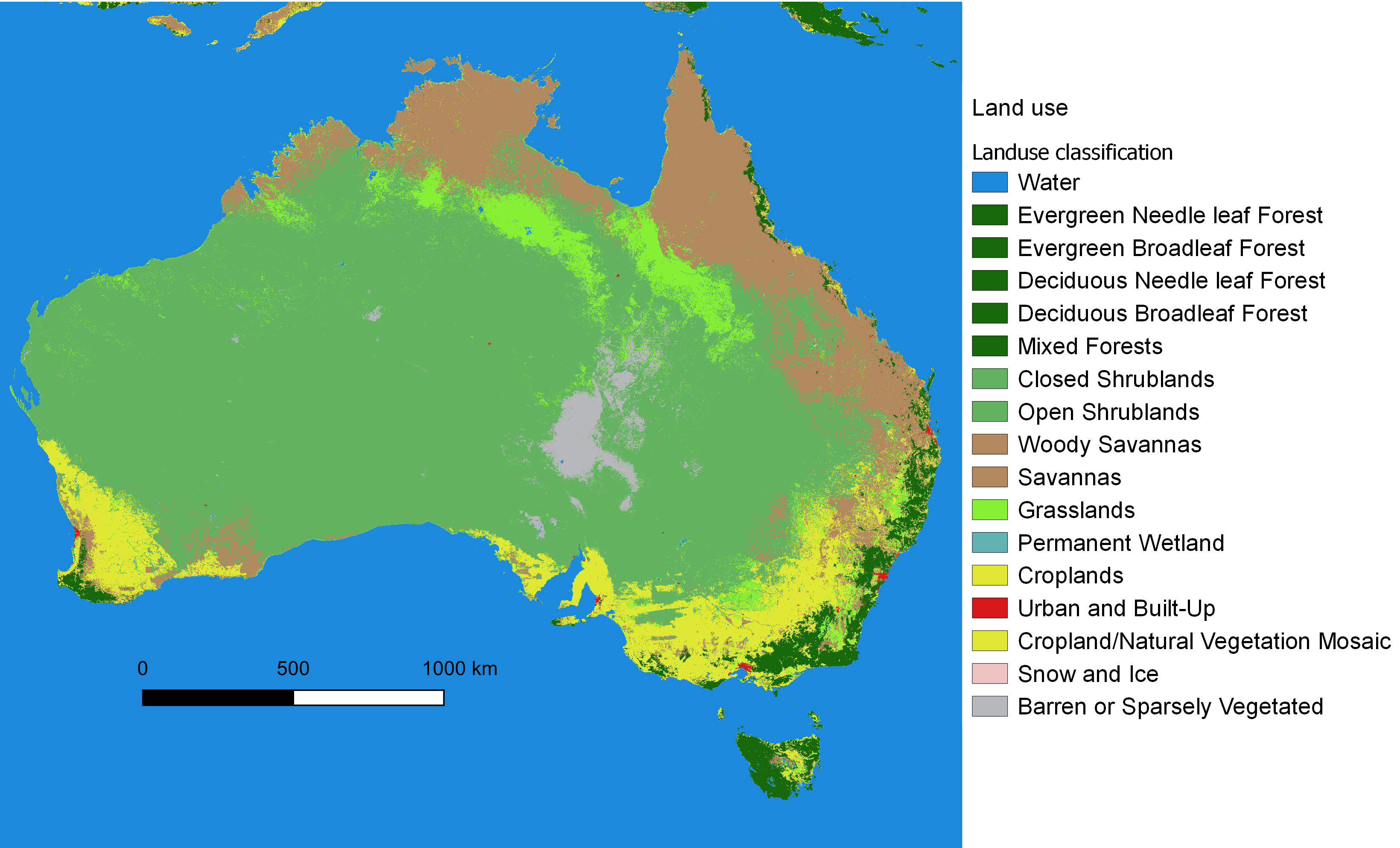
Land Use Raster Map - Australia¶
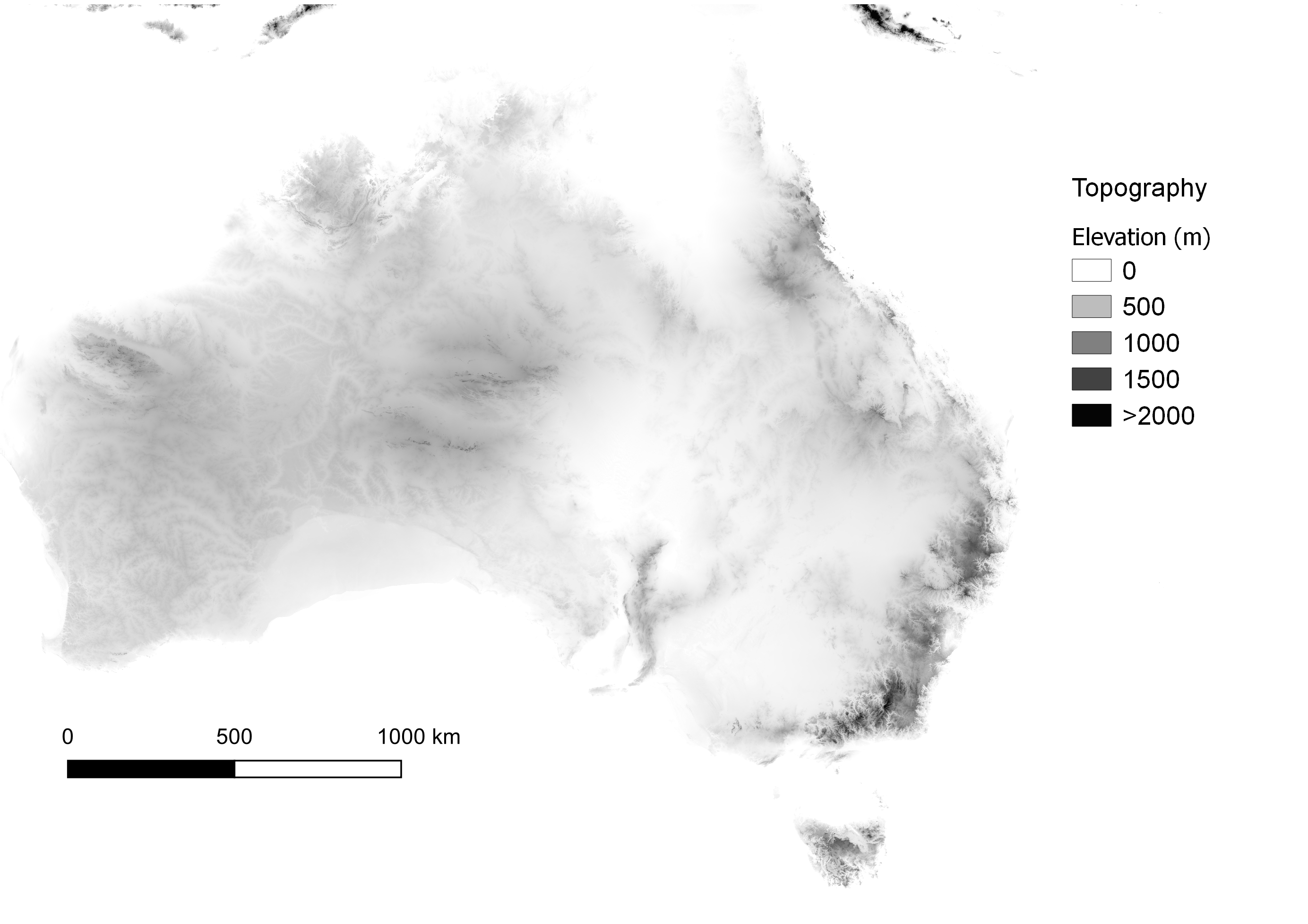
Topography Raster Map - Australia¶
Potential maps and reports¶
The lib.potential
module serves to generate potential raster maps for all four technologies supported by the script.
This module generates a Full-Load Hour (FLH) raster map, masking and masked rasters for unsuitable and protected areas,
and a weighting and weighted raster used for energy and power potential calculations.
It also generates a CSV report containing metrics for each subregion:
Available number of pixels, before and after masking
Available area in in km²
FLH mean, median, max, min values, before and after masking
FLH standard deviation after masking
Power Potential in GW, before and after weighting
Energy Potential in TWh in total, after weighting, and after masking and weighting
Sorted sample of FLH values for each region
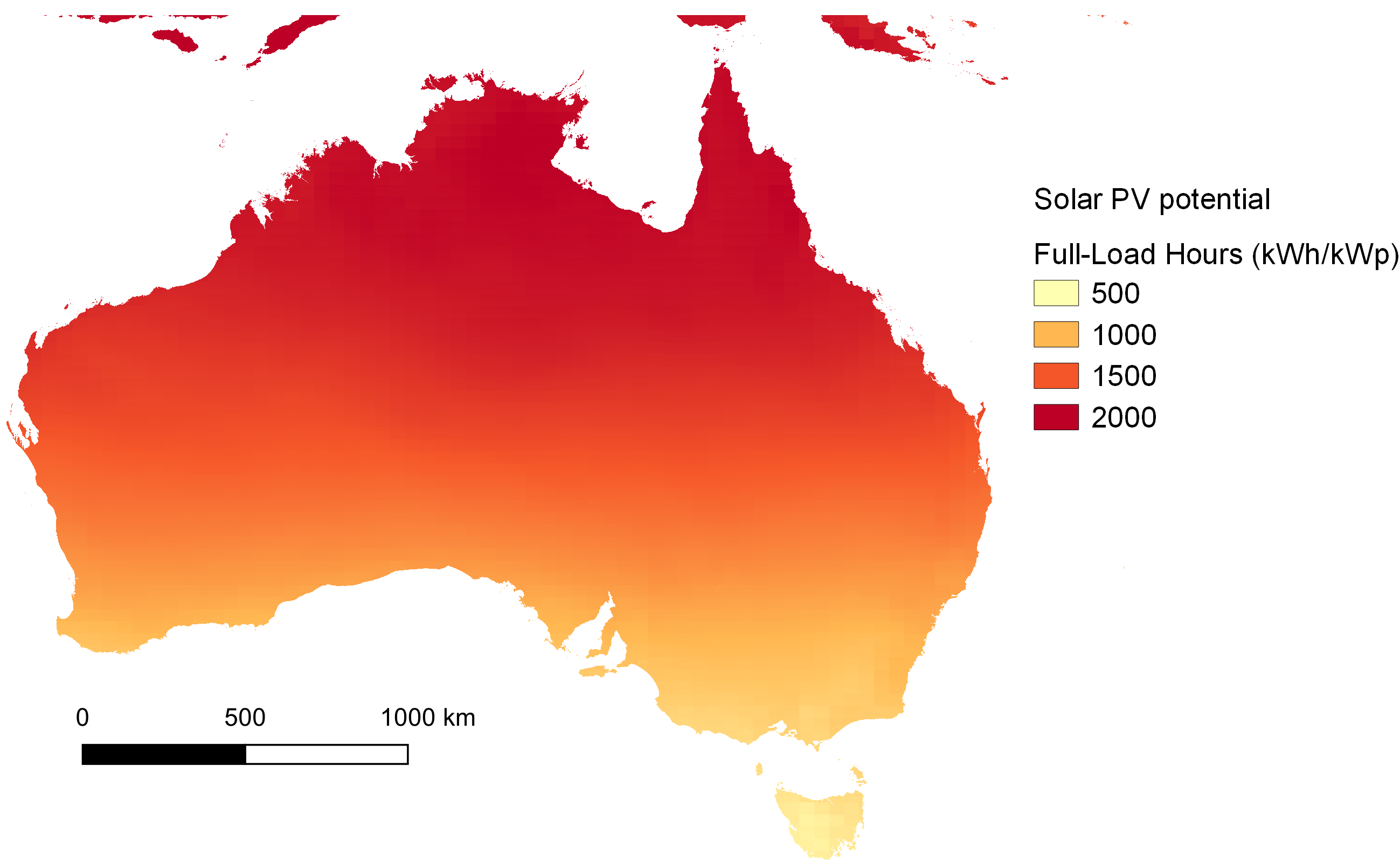
FLH of solar PV - Australia¶
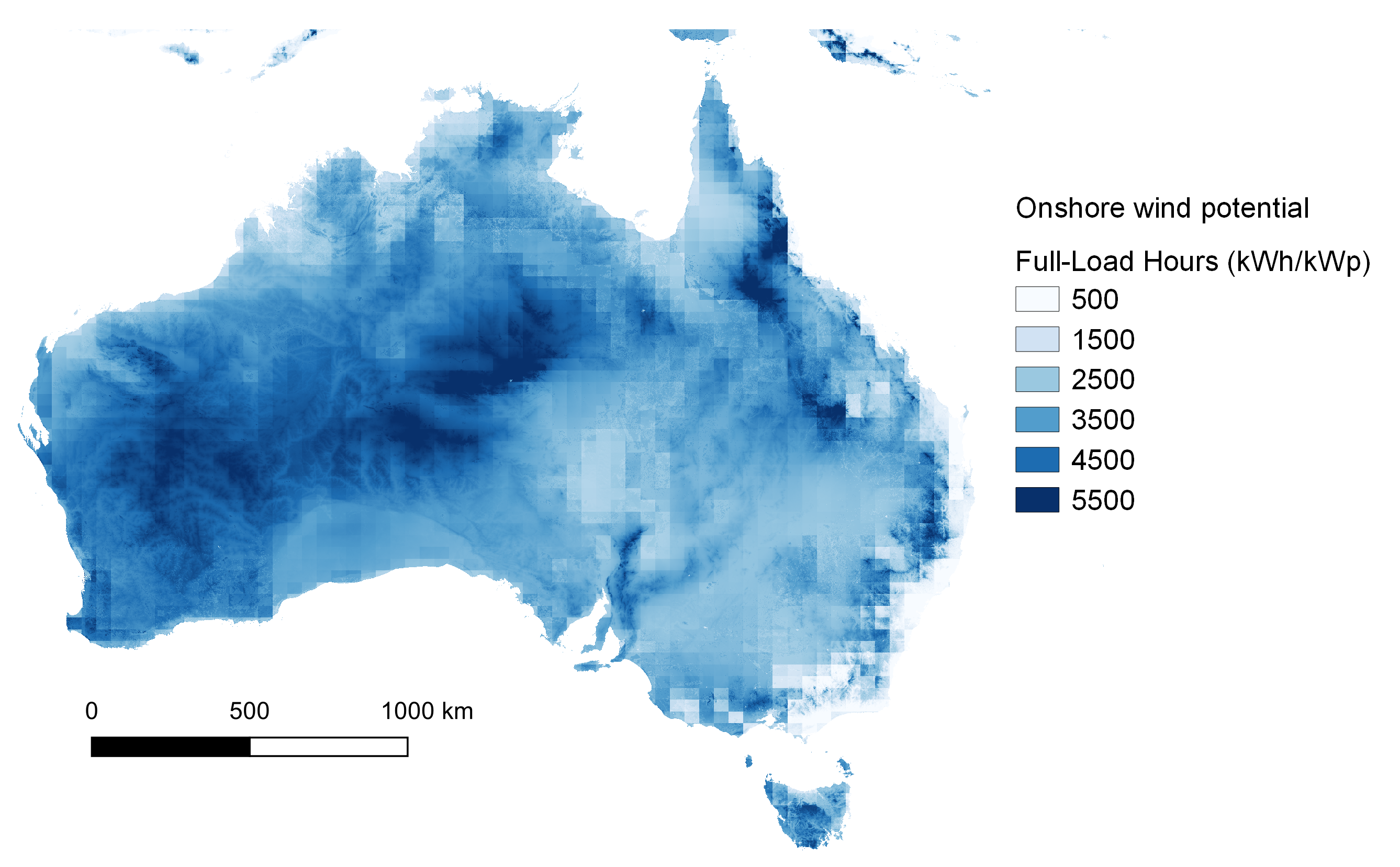
FLH of onshore wind - Australia¶
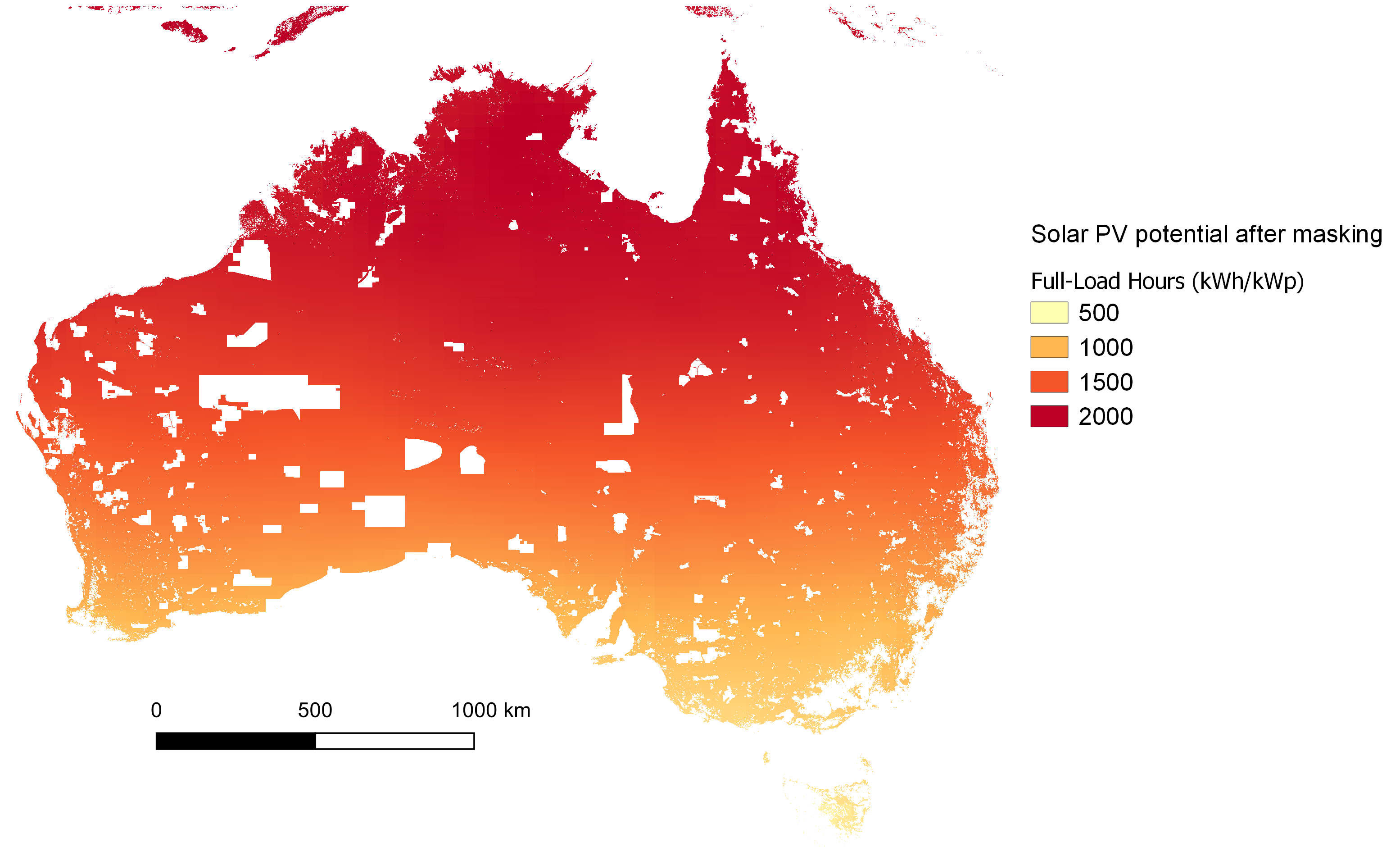
FLH of solar PV after masking - Australia¶
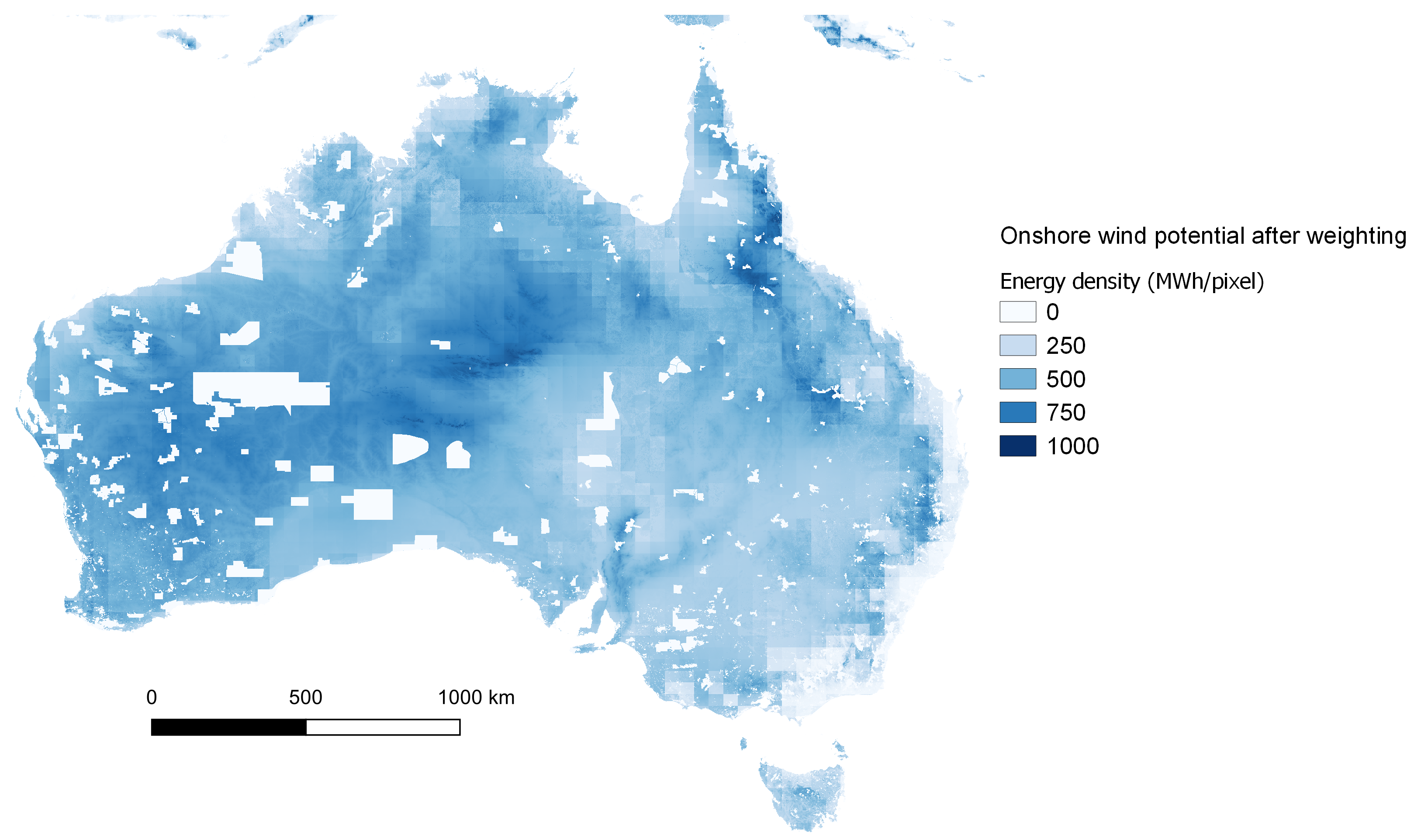
Energy output of onshore wind after weighting - Australia¶
Sample of potential report:
Region |
Available area (km²) |
---|---|
Region A |
4315.7 |
Region B |
2128.3 |
Region C |
561.3 |
Region D |
100953.1 |
Region E |
10.2 |
Region F |
2829.8 |
FLH mean |
FLH max |
FLH min |
Masked FLH mean |
Masked FLH max |
Masked FLH min |
Power potential (GW) |
Energy potential (TWh) |
---|---|---|---|---|---|---|---|
1638.4 |
1686.3 |
1578.0 |
1644.3 |
1686.3 |
1589.7 |
6.5 |
10.8 |
1682.9 |
1699.7 |
1601.6 |
1684.2 |
1695.0 |
1613.7 |
1.4 |
2.4 |
1849.7 |
1853.4 |
1833.8 |
1849.6 |
1853.3 |
1840.8 |
0.9 |
1.7 |
2017.6 |
2090.5 |
1986.8 |
2018.0 |
2086.1 |
1986.8 |
183.7 |
369.8 |
1856.8 |
1857.1 |
1856.5 |
1856.8 |
1857.1 |
1856.5 |
0.0 |
0.0 |
1729.5 |
1772.2 |
1659.1 |
1731.4 |
1772.2 |
1659.1 |
4.8 |
8.3 |
Time series for quantiles and user-defined locations¶
The lib.time_series
module allows to generate time series for quantiles as well as user-defined locations based on
the FLH raster maps generated in the previously mentioned module.
It is therefore important for the FLH raster maps to be generated first, in order to locate the quantiles.
However, generating time series for user-defined locations does not require the potential maps to be generated beforehand.

Wind Onshore and Solar PV capacity factor time series for quantile 50 - Australia¶
Regression¶
Once a set of time series for different settings (hub heights for wind technologies, orientations for solar PV) is generated,
the lib.regression
module allows the user to find a combination of settings and quantiles in order to match a known FLH value
and a given (typical) time series. The output is a set of regression coefficients that should be multiplied with the time series.
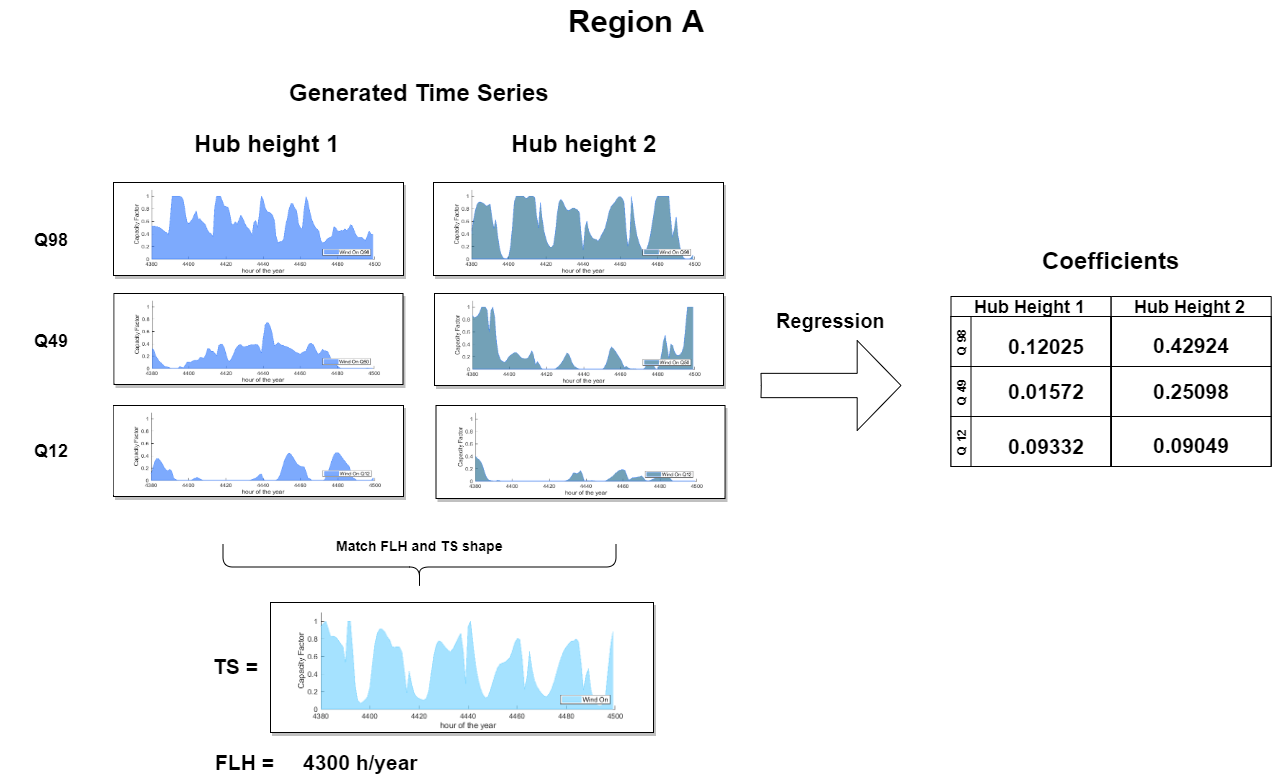
Regression Coefficients - Process example Region A¶
Stratified time series¶
Part of the lib.time_series
module, the lib.time_series.generate_time_series_for_regions
function reads the regression coefficients and
the generated time series, and combines them into user-defined modes (combinations of quantiles) and combos (combinations hub height or orientations settings).
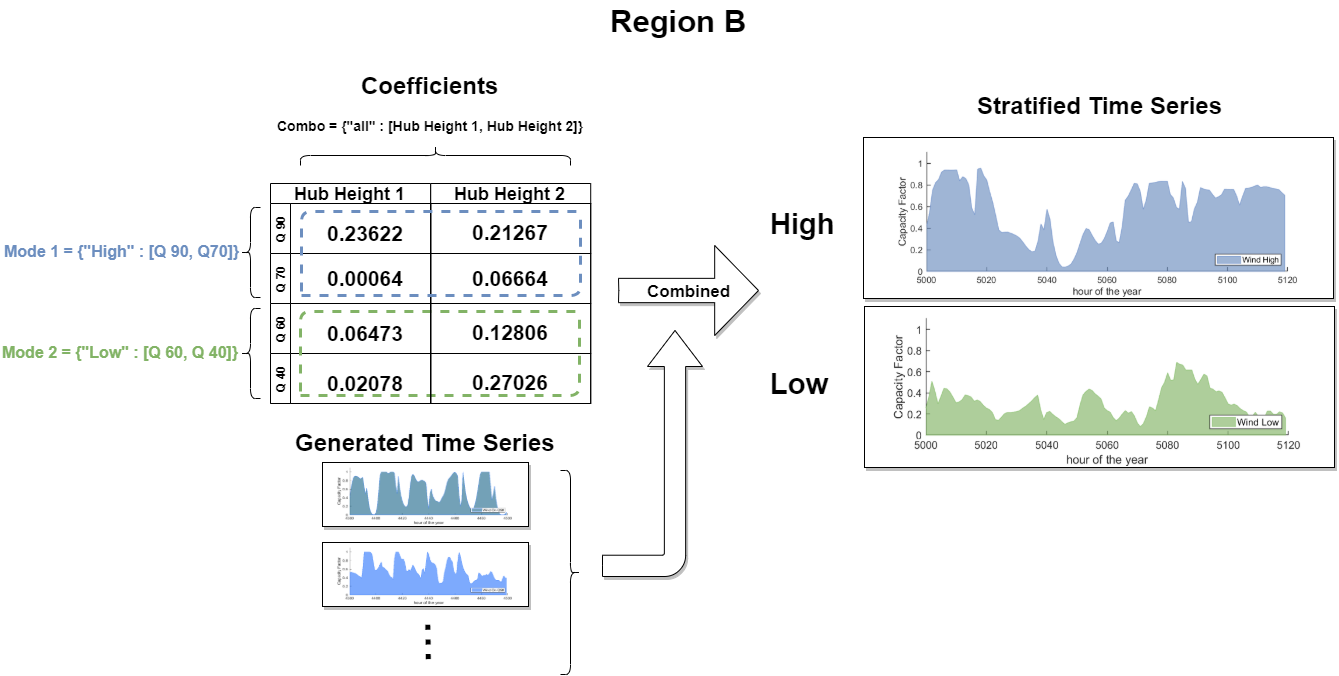
Stratified Time Series - Process example Region A¶
Example: - Graphic of Modes and Combos